一、下载 Spring
官方网站:http://projects.spring.io/spring-framework/ 下载方式:Maven、Gradle Maven 依赖描述:
1 2 3 4 5 6 7
| <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>4.3.2.RELEASE</version> </dependency> </dependencies>
|
二、Spring 概述
Spring 是一个开元框架,为简化企业级应用开发而生。使用 Spring 可以使简单的 JavaBean 实现以前只有 EJB 才能实现的功能。Spring 是一个 IOC 反转控制(DI 依赖注入)和 AOP(面向切面编程)容器框架。
- 轻量级:Spring 是非侵入性的 - 基于 Spring 开发的应用中对象可以不依赖 Spring 的 API;
- 依赖注入(DI, Dependency Injection、IOC);
- 面向切面编程(AOP, Aspect Oriented Programming);
- 容器:Spring 是一个容器,因为它包含并且管理应用对象的生命周期;
- 框架:Spring 实现了使用简单的组件配置组合成一个复杂的应用。在 Spring 中可以使用 XML 和 Java 注解组合这些对象;
- 一站式:在 IOC 和 AOP 的基础上可以整合各种企业应用的开源框架和优秀的第三方类库(实际上 Spring 自身也提供了表现层的 Spring MVC 和持久层的 Spring JDBC)。
三、Spring 模块
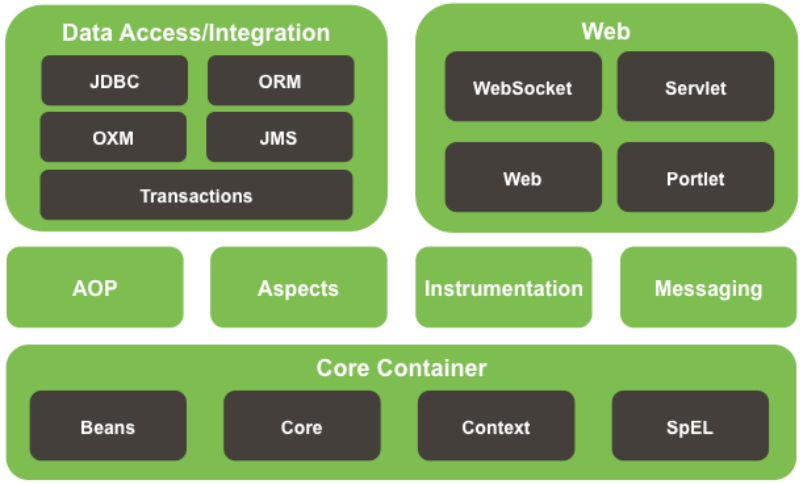
- SPRING TOOL SUITE 是一个 Eclipse 插件,利用该插件可以更方便的在 Eclipse 平台上开发基于 Spring 的应用。
- 安装方法说明(springsource-tool-suite-3.4.0.RELEASE-e4.3.1-updatesite.zip):
- Help –> Install New Software…
- Click Add…
- In dialog Add Site dialog, click Archive…
- Navigate to springsource-tool-suite-3.4.0.RELEASE-e4.3.1-updatesite.zip and click Open
- Clicking OK in the Add Site dialog will bring you back to the dialog ‘Install’
- Select the xxx/Spring IDE that has appeared
- Click Next and then Finish
- Approve the license
- Restart eclipse when that is asked
五、搭建 Spring 开发环境
- 将以下 jar 包加入到工程的 classpath 下:
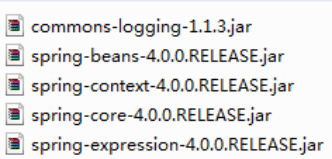
- Spring 的配置文件:一个典型的 Spring 项目需要创建一个或多个 Bean 配置文件,这些配置文件用于在 Spring IOC 容器中配置 Bean。Bean 的配置文件可以放在 classpath 下,也可以放在其它目录下。
六、创建 Spring 项目
- cn.javacodes.spring.beans.HelloWorld
1 2 3 4 5 6 7 8 9 10
| package cn.javacodes.spring.beans; public class HelloWorld { private String name; public void setName(String name){ this.name = name; } public void hello(){ System.out.println("hello: " + name); } }
|
- cn.javacodes.spring.beans.Main
1 2 3 4 5 6 7 8 9 10 11 12 13
| package cn.javacodes.spring.beans; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Main { public static void main(String[] args) { ApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml"); HelloWorld helloWorld = (HelloWorld) ctx.getBean("helloWorld"); helloWorld.hello(); } }
|
1 2 3 4 5 6 7 8 9
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!\-\- 配置Bean --> <bean id="helloWorld" class="cn.javacodes.spring.beans.HelloWorld"> <property name="name" value="Spring"></property> </bean> </beans>
|